Most Important topics: Map, Immutability and Synchronization
Thread vs runnable?
https://techdifferences.com/difference-between-thread-class-and-runnable-interface-in-java.html
Which algorithm java uses for sorting?
Tim sort for non primitives and Dual pivot quicksort for primitives.
b) Encapsulation : Encapsulation is similar to abstraction. It encapsulates data and code together and hide the implementation as necessary. In java, encapsulation is achieved through three access specifiers namely public, private and protected which provides four acess level, the fourth one being default. Also, in java everything has to be in class, so that binds the code and data together.
c) Inheritance : Inheritance is inheriting the properties and functionalities of a class thus providing code reuse. In java, we can inherit a class and/or multiple interfaces.
d) Polymorphism : Polymorphism is related to inheritance. polymorphism means 'many forms'. It is the ability of Java to identify the type of reference at runtime and call the appropriate method implemented by that object.It allows us to build flexible and maintainable system.
Thread vs runnable?
https://techdifferences.com/difference-between-thread-class-and-runnable-interface-in-java.html
Which algorithm java uses for sorting?
Tim sort for non primitives and Dual pivot quicksort for primitives.
What is OOPS ?
OOPS stand for Object Oriented Programming System. It's a programming paradigm where entire software system is composed of objects which interacts with each other and achieve a functionality. Each object has state and behaviour which allows this system to achieve dynamic features. OOPS has following four pillars:
a) Abstraction : Abstraction is hiding complexity. Abstraction allows us to focus on the idea and leave out the details. It helps in designing and creating architecuture of the application. In java, abstraction is achieved via Interfaces and abstract classes.OOPS stand for Object Oriented Programming System. It's a programming paradigm where entire software system is composed of objects which interacts with each other and achieve a functionality. Each object has state and behaviour which allows this system to achieve dynamic features. OOPS has following four pillars:
b) Encapsulation : Encapsulation is similar to abstraction. It encapsulates data and code together and hide the implementation as necessary. In java, encapsulation is achieved through three access specifiers namely public, private and protected which provides four acess level, the fourth one being default. Also, in java everything has to be in class, so that binds the code and data together.
c) Inheritance : Inheritance is inheriting the properties and functionalities of a class thus providing code reuse. In java, we can inherit a class and/or multiple interfaces.
d) Polymorphism : Polymorphism is related to inheritance. polymorphism means 'many forms'. It is the ability of Java to identify the type of reference at runtime and call the appropriate method implemented by that object.It allows us to build flexible and maintainable system.
How hashmap works in Java?
Hashmap works in Java using array and principle of hashing. Hashmap is used to store key value pairs. Internally it uses array to store them. In java , hashmap has an inner class called Entry which contains both key and value. When a new hashmap is created , an array of linkedlist is allocated internally , (if size is not speicified default 16). The linkedlist will hold Entry objects. Now , to use a class in hashmap as key , value it has to override equals and hashcode method properly. So , when a key and value pair is added to hashmap , it uses the hash value returned by hashcode method on key to determine the location (called bucket) in the array. It attached this entry to the linkedlist in that bucket.
When we try to fetch the value for that key, hashmap will again calculate bucket location using hash provided by hashcode method and traverse and search the linkedlist of entries. It it founds the key using equals method it returns the value.
Hashmap works in Java using array and principle of hashing. Hashmap is used to store key value pairs. Internally it uses array to store them. In java , hashmap has an inner class called Entry which contains both key and value. When a new hashmap is created , an array of linkedlist is allocated internally , (if size is not speicified default 16). The linkedlist will hold Entry objects. Now , to use a class in hashmap as key , value it has to override equals and hashcode method properly. So , when a key and value pair is added to hashmap , it uses the hash value returned by hashcode method on key to determine the location (called bucket) in the array. It attached this entry to the linkedlist in that bucket.
When we try to fetch the value for that key, hashmap will again calculate bucket location using hash provided by hashcode method and traverse and search the linkedlist of entries. It it founds the key using equals method it returns the value.
Difference between hashmap, hashtable and ConcurrentHashMap?
Hashmap and hashtable are exactly same except that all the methods of hashtable are synchronized. Also, hashmap allows one null key and multiple null values whereas hashtable doesn't allow null values. Hashtable is now a legacy class and it shouldn't be used. Hashtable used to implement Dictionary interface.
Hashmap and hashtable are exactly same except that all the methods of hashtable are synchronized. Also, hashmap allows one null key and multiple null values whereas hashtable doesn't allow null values. Hashtable is now a legacy class and it shouldn't be used. Hashtable used to implement Dictionary interface.
What will happen if I put a key which already exists in hashmap?
If we put a value to a key which already exists that value will simply override the already existing value.
Write your own implementations of hashmap.
Can arraylist be used instead of array?
If we put a value to a key which already exists that value will simply override the already existing value.
Write your own implementations of hashmap.
Can arraylist be used instead of array?
What is an interface?
Interface is an abstraction of behaviour which acts a contract in java, the implementing class has to implement all the methods declared in interface.
Difference between interface and abstract class? When to use which?
Abstract class can be used where a lot of classes have a common basic behaviour which can be abstracted in an abstract class otherwise interface should be used.
Interface is an abstraction of behaviour which acts a contract in java, the implementing class has to implement all the methods declared in interface.
Difference between interface and abstract class? When to use which?
Interface | Abstract Class |
---|---|
An interface can contain only declarations (though in java 8 interface can contain definition of a single method) | An abstract class con contain both declarations and definitions of methods. |
You can implement multiple interfaces in a class. | You can extend only one abstract class. |
Theoretically, interface is slower than abstract class though in modern times this isn't a big difference anymore. | Theoretically, abstract class is faster than interface. |
Overloading vs overriding?
Overloading | Overriding |
---|---|
Overloading refers to the feature that we can have multiple methods of same name but different arguments in a same class. | Overriding refers to the feature that a subclass can redefine the method defined in superclass. |
Overloading happens in same class | overriding happens in two different classes |
In overloading only method name is same and arguments must be different either in number or type. | In overriding , method name ,arguments and return type(except covariant return types) must exactly match. |
Why string is immutable?
String immutability offers a number of benefits:
a) String Pooling to save memory: In java, string pooling is used to save memory. It wouldn't have been possible if string weren't immutable.
b) Performance in map: String immutability makes them preferred choice for using them as keys in hashmap. It also provides better performance as hashmap can cache the hashcode value.
c) Security: It provides security because everything in java is done using string such as class name which is loaded by classloaders.
String immutability offers a number of benefits:
a) String Pooling to save memory: In java, string pooling is used to save memory. It wouldn't have been possible if string weren't immutable.
b) Performance in map: String immutability makes them preferred choice for using them as keys in hashmap. It also provides better performance as hashmap can cache the hashcode value.
c) Security: It provides security because everything in java is done using string such as class name which is loaded by classloaders.
How to make a class immutable?
A class can be made immutable by following rules:
a) Make the class final.
b) Make all fields private and don't provide any setters or public methods to modify them.
c) Make all fields final. So that we don't change them by mistake.
c) If class contains any other mutable class object, either make that class also immutable or don't provide it's reference. If the object is passed through constructor, don't assign it instead create a copy of it.
d) If class is returning a list, return an unmodifiable list using Collections.unmodifiablelist(list) or return a deep copy of that.
A class can be made immutable by following rules:
a) Make the class final.
b) Make all fields private and don't provide any setters or public methods to modify them.
c) Make all fields final. So that we don't change them by mistake.
c) If class contains any other mutable class object, either make that class also immutable or don't provide it's reference. If the object is passed through constructor, don't assign it instead create a copy of it.
d) If class is returning a list, return an unmodifiable list using Collections.unmodifiablelist(list) or return a deep copy of that.
What is difference between arraylist and linkedlist?
a) An arraylist is implemented using array whereas linkedlist is implemented using a linkedlist.
b) Arrays are ideal for fast lookup and traversing and slow for insertion and deletion. Linkedlist are ideal for lot of insertion and deletion and slow for lookup.
a) An arraylist is implemented using array whereas linkedlist is implemented using a linkedlist.
b) Arrays are ideal for fast lookup and traversing and slow for insertion and deletion. Linkedlist are ideal for lot of insertion and deletion and slow for lookup.
What is the difference between arraylist and vector?
a) Arraylist and vector are similar except that all the methods of Vector class are synchronized.
b)When arraylist is about to full it's size is increased to 50% of current capacity whereas vector is doubled.
a) Arraylist and vector are similar except that all the methods of Vector class are synchronized.
b)When arraylist is about to full it's size is increased to 50% of current capacity whereas vector is doubled.
What is difference between JDK, JRE and JVM?
JVM is the virtual machine which executes the bytecode of a java program.
JVM+system classes to run a program is called JRE. So, JRE is superset of JVM.
JRE+Debugger and other tools to assist a developer to write code is JDK(Java Development Kit). So, JDK is superset of JRE.
Why is JVM called virtual ?
JVM is called virtual because it's just a process but it acts like a machine and has PC(Program Counter), register ,etc.
JVM is the virtual machine which executes the bytecode of a java program.
JVM+system classes to run a program is called JRE. So, JRE is superset of JVM.
JRE+Debugger and other tools to assist a developer to write code is JDK(Java Development Kit). So, JDK is superset of JRE.
Why is JVM called virtual ?
JVM is called virtual because it's just a process but it acts like a machine and has PC(Program Counter), register ,etc.
Why use getters and setters?
Using getters and setters is a good coding practice as it provides encapsulation. There may be situation when we may have to edit and do some calculation or verification , it can be done in getters and setters . There may be situation where we may want to provide only read only permission.
Using getters and setters is a good coding practice as it provides encapsulation. There may be situation when we may have to edit and do some calculation or verification , it can be done in getters and setters . There may be situation where we may want to provide only read only permission.
Does Java support multiple inheritance? Why or why not?
Java supports multiple inheritance through interfaces only. A class can implement any number of interfaces but can extend only one class.
Multiple inheritance is not supported because it leads to deadly diamond problem. However , it can be solved but it leads to complex system so multiple inheritance has been dropped by Java founders.
In a white paper titled “Java: an Overview” by James Gosling in February 1995 gives an idea on why multiple inheritance is not supported in Java.According to Gosling: "JAVA omits many rarely used, poorly understood, confusing features of C++ that in our experience bring more grief than beneļ¬t. This primarily consists of operator overloading (although it does have method overloading), multiple inheritance, and extensive automatic coercions."
More info:
Stackoverflow
What are features of Java?
Java has a lot of features. As described by James Gosling in this whitepaper: "Java: An Overview".
1. Simple : Java has been kept very simple by omitting complicated concepts of C++ like operator overloading, multiple inheritance, manual memory management. According to Gosling, another aspect of simple is small. Java has been designed to run on small stand alone systems using very little memory.
2. Object Oriented : Java is fully Object Oriented except use of primitives, for which also it has provided wrapper classes. Java follows all the principles of OOP namely Abstraction, Encapsulation, Inheritance and Polymorphism.
3. Distributed : Quoting Gosling from the whitepaper : "JAVA now has a very extensive library of routines for easily coping with TCP/IP protocols like http and ftp. JAVA applications can open and access objects across the net via URLs with the same ease the programmers are used to accessing a local file system."
4. Robust : Java uses automatic memory management which removes the possiblity of a lot of bugs. Java doesn't use pointers. There is a strong exception handling mechanism and type checking mechanism. All these features make it very robust.
More info:
Type checking mechanism in Java
5. Secure : There is a strong interplay between robust and secure. Following features make Java secure language:
a) Java doesn't user pointers.
b) Java programs run inside virtual machine sandbox.
c) Bytecode verification
d) Secure class loading
6. Architecture-Neutral : Irrespective of type of underlying platform Java behaves in a same manner e.g, size of int
7. Portable : Java is platform-independent. This is due to use of Java bytecode and JVM. Java is first compiled to bytecodes which is then executed by JVM. There are different JVMs for different platform. However, there is no need of compiling Java program for different JVMs. Once compiled a Java program will run on any JVM.
8. Interpreted : Java is an interpreted language. The JVM interpretes the bytecode stream. It leads to better debugging and helps in making Java a dynamic language.(?)
9. High Performance: Java gives adequate performance. With the advent of JIT(Just in time compiler) the performance has improved even more.
10. Multi-threaded: Java supports concurrency and has a lot of in-built mechanisms to support concurrency.
11. Dynamic: Java is a dynamic language. Java implements OO paradigm. By coding to interface the behaviour of code is determined at runtime. One can easily add new classes, methods, etc. and change the implementation easily. Java also provide reflection.
What are the types of Exceptions ? How are they different?
There are two types of exceptions :
1) Checked Exception: These exceptions must be caught otherwise compiler will give error. All exceptions which extend Exception class except Runtime Exception and it's subclasses are checked exceptions. Checked exceptions represents conditions which are not in program control and can't do much about it such as file not found or the format of input is wrong. e.g, FileNotFoundException , ClassNotFoundException , ThreadInterruptedException , IOException
There are two types of exceptions :
1) Checked Exception: These exceptions must be caught otherwise compiler will give error. All exceptions which extend Exception class except Runtime Exception and it's subclasses are checked exceptions. Checked exceptions represents conditions which are not in program control and can't do much about it such as file not found or the format of input is wrong. e.g, FileNotFoundException , ClassNotFoundException , ThreadInterruptedException , IOException
2) Unchecked Exception : These exceptions needn't be caught.These reflect error in program logic from which program can't recover itself at runtime e.g, NullPointerException, ClassCastException, ArrayIndexOutOfBoundException, IllegalArgumentException, etc.
Can we override private, static methods?
No, we cannot override private and static methods because they are not inherited. However, we can redefine them in the subclass.
No, we cannot override private and static methods because they are not inherited. However, we can redefine them in the subclass.
What is generics, why use generics? Give an example.
Generics is a way to write code which can work with different types. It has many benefits:
1) Provides compile time safety.
2) Write generic algorithms
3) Saves us from casting objects
Generics is a way to write code which can work with different types. It has many benefits:
1) Provides compile time safety.
2) Write generic algorithms
3) Saves us from casting objects
A generic method to add two numbers:
public class GenericMethodToAdd { static <T extends Number> double add(T a,T b){ return a.doubleValue()+b.doubleValue(); } public static void main(String[] args) { System.out.println(add(1,2)); } }
Why can't we use polymorphism in generics?
We can't use polymorphism in generics because of type erasure. At runtime, all the information regarding parameter types is lost thus while adding something to collection JVM has no idea whether it's the same type or not. In case of arrays, JVM has the knowledge of the type, so it will throw ArrayStoreException.
We can't use polymorphism in generics because of type erasure. At runtime, all the information regarding parameter types is lost thus while adding something to collection JVM has no idea whether it's the same type or not. In case of arrays, JVM has the knowledge of the type, so it will throw ArrayStoreException.
What is a thread?
A thread is an independent path of execution in a program.
What is a process?
A process is a program in execution.
What's the difference between a thread and a process?
??Data Segment
Thread have direct access to the data segment of its process, an individual process has their own copy of the data segment of the parent process. See the difference between Stack and heap for more details.??
What is final keyword? How it's used in java?
Final keyword in java can be used with following:
Variable : When used with a primitive variable it makes it constant , i.e, it's value can't be changed ever.
Reference : When used with a reference, that reference can't point to some other object. However, the object can change it's content.
Method : When used with a method, the method can't be overridden.
Class : When used with a class, the class can't be subclassed.
Parameter : When used with a parameter, it has same meaning using with primitive variable or reference.
A thread is an independent path of execution in a program.
What is a process?
A process is a program in execution.
What's the difference between a thread and a process?
Thread | Process |
---|---|
Threads share same memory space. | Each process has it's own memory space. |
Because of above point, inter-thread communication becomes easy | Because of above point, inter-process communication is not so straightforward. |
Thread is lightweight | Process is heavyweight. |
Since thread is lightweight, context-switching is faster in case of threads. | Since process is heavyweight, context-switching is slower in case of processes. |
Since thread is lightweight, creating new threads is easy | Since process is heavyweight, creating new process consumes a lot of resources. |
Thread is part of a process. | Process can exist on it's own and can contain a number of threads. |
Changes to the parent thread like stop,start,priority,daemon,etc. can affect child threads. | Changes to the parent process doesn't affect child process. |
What is final keyword? How it's used in java?
Final keyword in java can be used with following:
Variable : When used with a primitive variable it makes it constant , i.e, it's value can't be changed ever.
Reference : When used with a reference, that reference can't point to some other object. However, the object can change it's content.
Method : When used with a method, the method can't be overridden.
Class : When used with a class, the class can't be subclassed.
Parameter : When used with a parameter, it has same meaning using with primitive variable or reference.
What's the importance of serializableversionid?
Serializableversionid is used to maintain the version of class. If we don't provide it explicitly, JVM internally provides one which it keeps on changing on every change to the class. Now, when we serialize and object, that serializableversionid is also saved. Now, when that object is deserialized, it's serializableversionid is matched with the id of the class. If there is a mismatch an 'InvalidClassException' is thrown.
Serializableversionid is used to maintain the version of class. If we don't provide it explicitly, JVM internally provides one which it keeps on changing on every change to the class. Now, when we serialize and object, that serializableversionid is also saved. Now, when that object is deserialized, it's serializableversionid is matched with the id of the class. If there is a mismatch an 'InvalidClassException' is thrown.
What if I put return in try block, catch block and finally block?
Finally block is always executed even if we put return statement in try or catch block. The value returned by the finally block will override the value returned earlier.
In which scenario finally is not executed?
Finally is not executed if JVM shuts down or System.exit() is called from the code.
Finally block is always executed even if we put return statement in try or catch block. The value returned by the finally block will override the value returned earlier.
In which scenario finally is not executed?
Finally is not executed if JVM shuts down or System.exit() is called from the code.
What is garbage collection? How it's done?
The automatic reclaimation of memory from objects which are no longer in use is called garbage collection.
The automatic reclaimation of memory from objects which are no longer in use is called garbage collection.
Garbage collection is done by a continously running daemon thread. How it's scheduled and when it will run is totally unpredictable and depend on JVM, however it will surely run if the application is running out of memory. Garbage collection runs on various algorithms. Based on the type of machine the garbage collector is chosen by the JVM. Usually parallel GC is the default one.
Java memory is divided mainly into two parts, heap and stack. All the local variables and function definition goes on stack, rest go on heap such as objects, static variables, etc. In java 8, heap is further divided into young,old and tenured. The short lived objects resides in young. Initially, all objects go to young, those who survive garbage collection are then moved to old and finally tenured. Garbage collection happens more often in young heap area. In Java 8, permgen area has also been replaced with metadata. Now, string pool is not limited in size, and users won't get permgen out of memory error.
What are types of garbage collectors in java?
Java has four types of garbage
collectors,
Serial Garbage Collector
Parallel Garbage Collector
CMS Garbage Collector
G1 Garbage Collector
Serial
garbage collector works by holding all the application threads. It is designed
for the single-threaded environments. It uses just a single thread for garbage
collection. The way it works by freezing all the application threads while
doing garbage collection may not be suitable for a server environment. It is
best suited for simple command-line programs.
Turn on the
-XX:+UseSerialGC
JVM argument to use the serial garbage
collector.
2. Parallel Garbage Collector
Parallel
garbage collector is also called as throughput collector. It is the default
garbage collector of the JVM. Unlike serial garbage collector, this uses
multiple threads for garbage collection. Similar to serial garbage collector
this also freezes all the application threads while performing garbage
collection.
3. CMS Garbage Collector
Concurrent
Mark Sweep (CMS) garbage collector uses multiple threads to scan the heap
memory to mark instances for eviction and then sweep the marked instances. CMS
garbage collector holds all the application threads in the following two
scenarios only,
- while marking the referenced
objects in the tenured generation space.
- if there is a change in heap memory
in parallel while doing the garbage collection.
In
comparison with parallel garbage collector, CMS collector uses more CPU to
ensure better application throughput. If we can allocate more CPU for better
performance then CMS garbage collector is the preferred choice over the
parallel collector.
Turn on the
XX:+USeParNewGC
JVM argument to use the CMS garbage collector.
4. G1 Garbage Collector
G1 garbage
collector is used for large heap memory areas. It separates the heap memory
into regions and does collection within them in parallel. G1 also does compacts
the free heap space on the go just after reclaiming the memory. But CMS garbage
collector compacts the memory on stop the world (STW) situations. G1 collector
prioritizes the region based on most garbage first.
Turn on the
–XX:+UseG1GC
JVM argument to use the G1 garbage collector.
Java 8 Improvement
Turn on the
-XX:+UseStringDeduplication
JVM argument while using G1 garbage collector.
This optimizes the heap memory by removing duplicate String values to a single
char[] array. This option is introduced in Java 8 u 20.
Given all
the above four types of Java garbage collectors, which one to use depends on
the application scenario, hardware available and the throughput requirements.
What is the use volatile, transient keywords?
Volatile keyword is used in multi-threading environment. It can only be used with variables. A volatile is variable is always read from main memory. Thus, it enforces happens-before-relationship i.e, if a thread updates the variable value when another thread will read it, it will see the updated value. One important point to note is that not only that variable but all the variables which were seen by the thread updating the variable will follow the happens-before-relationship.
Volatile keyword is used in multi-threading environment. It can only be used with variables. A volatile is variable is always read from main memory. Thus, it enforces happens-before-relationship i.e, if a thread updates the variable value when another thread will read it, it will see the updated value. One important point to note is that not only that variable but all the variables which were seen by the thread updating the variable will follow the happens-before-relationship.
What is java memory model?
Java memory model describes the relationship of threads with memory. It defines the happens before relationship contracts. If there is a single thread then if statement x comes before statement y, there is happens before relationship such that x happens before y. A volatile write on a variable happens before a subsequent read on that variable. An unlock on an object will happens before subsequent lock on that object and all changes made in a synchronized block will happens before (and thus will be seen by all other threads) before subsequent lock on the same object. Similar happens before relationship contracts exits for other things such as Atomic variables, AtomicInteger, AtomicLong, etc.
Java memory model describes the relationship of threads with memory. It defines the happens before relationship contracts. If there is a single thread then if statement x comes before statement y, there is happens before relationship such that x happens before y. A volatile write on a variable happens before a subsequent read on that variable. An unlock on an object will happens before subsequent lock on that object and all changes made in a synchronized block will happens before (and thus will be seen by all other threads) before subsequent lock on the same object. Similar happens before relationship contracts exits for other things such as Atomic variables, AtomicInteger, AtomicLong, etc.
String vs StringBuilder?
a) String is an immutable class whereas StringBuilder is mutable.
c) StringBuilder provides some additional methods such as append,reverse.
d) StringBuilder doesn't override equals method.
StringBuilder vs StringBuffer
a) String is an immutable class whereas StringBuilder is mutable.
c) StringBuilder provides some additional methods such as append,reverse.
d) StringBuilder doesn't override equals method.
StringBuilder vs StringBuffer
What is thread safety?
Thread safety refers to making your code immune to problems occuring due to concurrent access of resources by multiple threads.
What is race condition?
When two or more than two threads are trying to modify/access a shared resource, it is called race condition.
What is deadlock?
Deadlock is a state when a program has halted due to two or more threads trying to acquire resources held by each other.
What are the conditions for deadlock?
There are four conditions for a deadlock to happen:
a) Circular dependencies
b) Non-preemptive
c) Mutual exclusion
d) Hold and wait(?)
Give an example of deadlock.
Four cars deadlock:
How to prevent deadlock?
Deadlock can be prevented if threads acquire resources in same order.
What is livelock?
Livelock is the situation when two threads are giving up their resources to let other thread progress, however none of them progressing. A good example is when two persons are trying to cross each other in a narrow corridor and give each other way to pass and none of them able to cross each other.
Semaphore vs mutex vs lock vs synchronized?
Semaphore is like counting resources.
Mutex is mutual exclusion.
Semaphore with count 1 can be used as lock and mutex.(?)
Lock is same as mutex.
Synchronized is allowing only one thread to access some code. It can be achieved by various ways, using synchronized keyword, using mutex or lock.
Semaphore is like counting resources.
Mutex is mutual exclusion.
Semaphore with count 1 can be used as lock and mutex.(?)
Lock is same as mutex.
Synchronized is allowing only one thread to access some code. It can be achieved by various ways, using synchronized keyword, using mutex or lock.
import java.util.concurrent.Semaphore; public class SemaphoreDemo { public void read(Semaphore semaphore) throws Exception{ semaphore.acquire(); System.out.println(Thread.currentThread().getName()+" reading"); Thread.sleep(2000); System.out.println(Thread.currentThread().getName()+" finished"); semaphore.release(); } public static void main(String[] args) { final Semaphore semaphore = new Semaphore(3); final SemaphoreDemo demo = new SemaphoreDemo(); for(int i=1;i<=5;i++){ Thread t = new Thread(new Runnable() { @Override public void run() { try { demo.read(semaphore); } catch (Exception e) { e.printStackTrace(); } } }); t.start(); } } }
What is classloader? Types? How classloader works?
Classloader loads classes in memory. There are three types of classloaders:
a) Bootstrap Classloader: It loads the classes at JVM start. It loads the rt.jar.
b) Extension Classloader: It loads the classes which extends JVM funtionality. It loads the jar in lib/ext directory.
c) System Classloader: It loads the classes defined by classpath. It can be extended by user to create custom classloader.
Classloaders are heirarchical in nature, every classloader first checks with it's parent before trying to load a class by itself. Only parents can see the the classes loaded by it's children.
Classloader loads classes in memory. There are three types of classloaders:
a) Bootstrap Classloader: It loads the classes at JVM start. It loads the rt.jar.
b) Extension Classloader: It loads the classes which extends JVM funtionality. It loads the jar in lib/ext directory.
c) System Classloader: It loads the classes defined by classpath. It can be extended by user to create custom classloader.
Classloaders are heirarchical in nature, every classloader first checks with it's parent before trying to load a class by itself. Only parents can see the the classes loaded by it's children.
25. What is JVM? How does it works? Is it platform independent?JVM stands for Java Virtual Machine. JVM has three notions:
Specification : A document describing the overview of JVM. It's the abstract form of JVM. This specification ensures all the JVMs are interoperable. It omits the low level implementation specific of JVM such as memory model , garbage collection algorithm , internal optimization of JVM , etc.
Implementation : Implementation of specification of JVM. e.g. HotSpot
Instance : Implementation of JVM currently running on a machine as a process.
No, JVM is not platform independent. There is specific JVM for each platform and this is the way how Java is platform independent.
Specification : A document describing the overview of JVM. It's the abstract form of JVM. This specification ensures all the JVMs are interoperable. It omits the low level implementation specific of JVM such as memory model , garbage collection algorithm , internal optimization of JVM , etc.
Implementation : Implementation of specification of JVM. e.g. HotSpot
Instance : Implementation of JVM currently running on a machine as a process.
No, JVM is not platform independent. There is specific JVM for each platform and this is the way how Java is platform independent.
- JVM Architecture
Can I overload main method?
Yes
Yes
What is ThreadLocal class? Why use it?
This class provides thread-local variables. It is used to create thread-safe object without synchronizing them. ThreadLocal instances are usually private static fields in classes that wish to associate state with a thread such as userid, transactionid, datetime, etc.
Examples:
This class provides thread-local variables. It is used to create thread-safe object without synchronizing them. ThreadLocal instances are usually private static fields in classes that wish to associate state with a thread such as userid, transactionid, datetime, etc.
Examples:
public class Foo { // SimpleDateFormat is not thread-safe, so give one to each thread private static final ThreadLocal<SimpleDateFormat> formatter = new ThreadLocal<SimpleDateFormat>(){ @Override protected SimpleDateFormat initialValue() { return new SimpleDateFormat("yyyyMMdd HHmm"); } }; public String formatIt(Date date) { return formatter.get().format(date); } }
import java.util.concurrent.atomic.AtomicInteger; public class ThreadId { // Atomic integer containing the next thread ID to be assigned private static final AtomicInteger nextId = new AtomicInteger(0); // Thread local variable containing each thread's ID private static final ThreadLocal<Integer> threadId = new ThreadLocal<Integer>() { @Override protected Integer initialValue() { return nextId.getAndIncrement(); } }; // Returns the current thread's unique ID, assigning it if necessary public static int get() { return threadId.get(); } }
Wait vs sleep vs yield?
Wait gives up the lock.
Wait can be called only from inside synchronized block.
Wait will resume after it gets notified. There is timed waiting also, but there is not guarantee that thread will resume.
Sleep holds the lock and can be called from anywhere.
Sleep will resume after the particular period specified.
Wait gives up the lock.
Wait can be called only from inside synchronized block.
Wait will resume after it gets notified. There is timed waiting also, but there is not guarantee that thread will resume.
Sleep holds the lock and can be called from anywhere.
Sleep will resume after the particular period specified.
Yield temporarily stops running and enter the runnable state. It is used to give other threads a chance to execute.
Is java compiled language or interpreted language?
Both.
Java was basically an interpreted language. However, it made it very slow. A lot of optimizations have been made now and JIT has been introduced. The code which is frequenty accessed is compiled by the JVM to improve the performance.
Both.
Java was basically an interpreted language. However, it made it very slow. A lot of optimizations have been made now and JIT has been introduced. The code which is frequenty accessed is compiled by the JVM to improve the performance.
Executor.submit vs Executor.execute?
Executor.submit can return result and throw exception while Executor.execute only execute the task.
Executor.submit takes Callable as input and Executor.execute takes Runnable.
Executor.submit can return result and throw exception while Executor.execute only execute the task.
Executor.submit takes Callable as input and Executor.execute takes Runnable.
What are the major Java releases and their important features ?
Java 8 was released in March 2014. It is considered a major release since java 5. Following are the salient features of Java 8:
1. Lambda expressions- Anonymous functions
2. Functional Interface and default methods in interface
3. New Memory Model in which permanent generation space has been removed.
4.Streams , pipes and filters :For iterating over collection values
5. New Javascript engine Nashorn for integrating Javascript code
6. New Joda Date Time API
7. Concurrent Accumulators
8. Type Annotations, Repeating Annotations
Java 8 was released in March 2014. It is considered a major release since java 5. Following are the salient features of Java 8:
1. Lambda expressions- Anonymous functions
2. Functional Interface and default methods in interface
3. New Memory Model in which permanent generation space has been removed.
4.Streams , pipes and filters :For iterating over collection values
5. New Javascript engine Nashorn for integrating Javascript code
6. New Joda Date Time API
7. Concurrent Accumulators
8. Type Annotations, Repeating Annotations
Java 7 released in July ,2011. Java 7 added a few minor imporvements such as :
1. Strings can be used in switch expression.
2. Better Generic type inference in diamond operator
3. Underscores can be used in long numeric values for readability.
4. Automatic resource management in try
5. Multiple exceptions can be caught in single catch block.
1. Strings can be used in switch expression.
2. Better Generic type inference in diamond operator
3. Underscores can be used in long numeric values for readability.
4. Automatic resource management in try
5. Multiple exceptions can be caught in single catch block.
Java 6 released in December 2006 had minor improvements in various features. This version Java 2 was dropped and Java was renamed to JSE and JEE from J2SE and J2EE respectively.
Java 5 released in September 2004 was a revolutionary version upgrade for Java. It added many new features such as :
1. Generics
2. Var-args
3. AutoBoxing
4. Enhanced for each loop
5. Annotations
1. Generics
2. Var-args
3. AutoBoxing
4. Enhanced for each loop
5. Annotations
Java 2 released in Dec, '98 introduced Collections framework.
What are lambda expressions ?
Lambda expressions are nothing but anonymous functions.
Lambda expressions are nothing but anonymous functions.
Which sorting algorithm in used in Collections.sort() method?
For primitives it uses quicksort(unstable), for objects it uses tim sort(stable).
Till Java6 merge sort was used and since Java 7 it uses a modified merge sort called Tim sort. Tim sort guarantees time complexity of O(nlogn). It's a combination of merge sort and insertion sort. Read more here : https://www.wikiwand.com/en/Timsort
What do you mean by platform independence of Java?For primitives it uses quicksort(unstable), for objects it uses tim sort(stable).
Till Java6 merge sort was used and since Java 7 it uses a modified merge sort called Tim sort. Tim sort guarantees time complexity of O(nlogn). It's a combination of merge sort and insertion sort. Read more here : https://www.wikiwand.com/en/Timsort
Java is a platform independent language, java code once compiled can run on any platform such as Windows, Unix, Linux, etc. It achieves platform independence by compiling source code to a special form called bytecode which is then executed by JVM which is specific for each machine.
What is JRE ?
JVM + Java Class library is JRE.
JVM + Java Class library is JRE.
According to Oracle :
The Java Runtime Environment (JRE) provides the libraries, the Java Virtual Machine, and other components to run applets and applications written in the Java programming language. In addition, two key deployment technologies are part of the JRE: Java Plug-in, which enables applets to run in popular browsers; and Java Web Start, which deploys standalone applications over a network. It is also the foundation for the technologies in the Java 2 Platform, Enterprise Edition (J2EE) for enterprise software development and deployment. The JRE does not contain tools and utilities such as compilers or debuggers for developing applets and applications.
The Java Runtime Environment (JRE) provides the libraries, the Java Virtual Machine, and other components to run applets and applications written in the Java programming language. In addition, two key deployment technologies are part of the JRE: Java Plug-in, which enables applets to run in popular browsers; and Java Web Start, which deploys standalone applications over a network. It is also the foundation for the technologies in the Java 2 Platform, Enterprise Edition (J2EE) for enterprise software development and deployment. The JRE does not contain tools and utilities such as compilers or debuggers for developing applets and applications.
What is JDK ?
JRE + Java Compiler + Debugger and other tools to develop Java application is JDK.
According to Oracle : The JDK is a superset of the JRE, and contains everything that is in the JRE, plus tools such as the compilers and debuggers necessary for developing applets and applications.
JRE + Java Compiler + Debugger and other tools to develop Java application is JDK.
According to Oracle : The JDK is a superset of the JRE, and contains everything that is in the JRE, plus tools such as the compilers and debuggers necessary for developing applets and applications.
What is framework?
A framework is an environment/platform for developing an application. It provides reusable, generic functionality which can be specialized by the user. A framework brings together several components.
A framework is an environment/platform for developing an application. It provides reusable, generic functionality which can be specialized by the user. A framework brings together several components.
What is library?
A library is a set of API(Application Programming Interface) which provides universal, reusable functionality.
A library is a set of API(Application Programming Interface) which provides universal, reusable functionality.
What is the difference between library and framework ?
There are a number of differences between library and framework :
a) Inversion of Control : A framework controls the program's control of flow.
b) Default behaviour : A framework has a default behaviour whereas a library doesn't.
There are a number of differences between library and framework :
a) Inversion of Control : A framework controls the program's control of flow.
b) Default behaviour : A framework has a default behaviour whereas a library doesn't.
Which class is the superclass of all classes?
Object
Why Java doesn’t support multiple inheritance?
Java 8 does support multiple inheritance. Java doesn't support multiple inheritance because that would lead to diamond problem. But I think the Java developers decided not to support multiple inheritance because that leads to complex and rigid software.
Why Java is not pure Object Oriented language?
Java is not purely OOP because it has support for primitive variables.
Object
Why Java doesn’t support multiple inheritance?
Java 8 does support multiple inheritance. Java doesn't support multiple inheritance because that would lead to diamond problem. But I think the Java developers decided not to support multiple inheritance because that leads to complex and rigid software.
Why Java is not pure Object Oriented language?
Java is not purely OOP because it has support for primitive variables.
What is OOPS ?OOPS stand for Object Oriented Programming System. OOPS has four parts (which can be remembered by the acronym APIE):
- Abstraction : (explain these later)
- Polymorphism
- Inheritance
- Encapsulation
What is difference between path and classpath variables?
Path is where OS search for executables, classpath is where JVM searches for java classes.
What is overloading and overriding in java?
Overloading : When a class contains different functions with the same name but different number of arguments or different types of arguments than it is called overloading. It is also called compile time polymorphism.
Overriding : When a subclass redefines the same method defined in superclass then is called overriding. It is called dynamic polymorphism or just polymorphism.
Path is where OS search for executables, classpath is where JVM searches for java classes.
What is overloading and overriding in java?
Overloading : When a class contains different functions with the same name but different number of arguments or different types of arguments than it is called overloading. It is also called compile time polymorphism.
Overriding : When a subclass redefines the same method defined in superclass then is called overriding. It is called dynamic polymorphism or just polymorphism.
Can we overload main method?
Yes.
Yes.
Can we have multiple public classes in a java source file?
No.
Can we have multiple classes in a java source file?
Yes
No.
Can we have multiple classes in a java source file?
Yes
What is Java Package and which package is imported by default?
A package is a namespace for defining classes. By default , java.lang is imported.
A package is a namespace for defining classes. By default , java.lang is imported.
What are access modifiers?
There are three acess modifiers which provide four levels of acces in Java:
There are three acess modifiers which provide four levels of acces in Java:
- Public : Any class in any package can access a public variable or class.
- Private : Only members of same class can access private members.
- Protected : Only classes of same package and subclasses can access protected members.
- Default : This is not a keyword. If we don't use any keyword it means it has default access level. Only classes of the same package can access default members.
What is final keyword?
Final keyword when used with different Java element provides different functionality:
Final keyword when used with different Java element provides different functionality:
- Class : Can't be extended.
- Method : Can't be overriden.
- Primitive : Value can't be changed.
- Reference: Can't refer to some other object.
What is static keyword?
It makes the values, method or class static which means it will be associated with the class state and all the instances of that class will share that.
What is finally and finalize in java?
Finally block is always executed. It is used with try statement.
Finalize is a method which is called before garbage collecting the java object.
Can we declare a class as static?
Only inner classes can be declared static. These are then called static nested classes or just nested classes.
It makes the values, method or class static which means it will be associated with the class state and all the instances of that class will share that.
What is finally and finalize in java?
Finally block is always executed. It is used with try statement.
Finalize is a method which is called before garbage collecting the java object.
Can we declare a class as static?
Only inner classes can be declared static. These are then called static nested classes or just nested classes.
What is static import?
Static import was introduced in Java 5(?) , it allows you to use the static members of that class without specifying the class name.(However, keep in mind that usage is import static.)
Static import was introduced in Java 5(?) , it allows you to use the static members of that class without specifying the class name.(However, keep in mind that usage is import static.)
What is try-with-resources in java?
Try-with-resource was introduced in Java 7. Using this we don't need to explicitly close the resources opened in try with resource block. Here exception is final(??)
Try-with-resource was introduced in Java 7. Using this we don't need to explicitly close the resources opened in try with resource block. Here exception is final(??)
What is multi-catch block in java?
Java 7 introduced multi-catch block which is various execptions separated by a pipeline. It's functionality is almost similar to having multiple catch blocks. Or Here exception is final(??)
Java 7 introduced multi-catch block which is various execptions separated by a pipeline. It's functionality is almost similar to having multiple catch blocks. Or Here exception is final(??)
What is static block?
Static block is also called static initializer block. It runs when class is loaded.
Static block is also called static initializer block. It runs when class is loaded.
What is an interface?
An interface is an abstraction of behaviour which acts as a contract for the implementing class.
Can method be called from constructor?
Yes
What is the output of below code?
In Derived and i=10
What is an abstract class?
A class declared with the abstract keyword is called an abstract class. An abstract class cannot be instantiated and may contain abstract methods which are just declaration of methods.
An interface is an abstraction of behaviour which acts as a contract for the implementing class.
Can method be called from constructor?
Yes
What is the output of below code?
package general; class Base{ int i=10; Base(){ meth(); } void meth(){ System.out.println("In Base and i="+i); } } class Derived extends Base{ Derived(){ i=20; } void meth(){ System.out.println("In Derived and i="+ i); } } public class InheritancePuzzle { public static void main(String[] args) { Base b = new Derived(); } }
In Derived and i=10
What is an abstract class?
A class declared with the abstract keyword is called an abstract class. An abstract class cannot be instantiated and may contain abstract methods which are just declaration of methods.
What is method declaration and definition?
Declaration describes the method signature whereas definition describes the implementation of the method.
Declaration describes the method signature whereas definition describes the implementation of the method.
What is the difference between abstract class and interface?
Abstract class can contain generic default behaviour whereas interface contains only declaration of methods, although in Java 8 interfaces can have default implementation of methods.
Rest are other basic class vs interface
Abstract class can contain generic default behaviour whereas interface contains only declaration of methods, although in Java 8 interfaces can have default implementation of methods.
Rest are other basic class vs interface
Can an interface implement or extend another interface?
An Interface can extend other interfaces but can't implement other interfaces.
An Interface can extend other interfaces but can't implement other interfaces.
What is Marker interface?
An interface without any methods is called Marker interface such as Serialization , Cloneable, Random Access, etc.
An interface without any methods is called Marker interface such as Serialization , Cloneable, Random Access, etc.
What are Wrapper classes?
These are the classes which wraps around the primitive to give them behaviour of an object. All wrapper classes are immutable.
These are the classes which wraps around the primitive to give them behaviour of an object. All wrapper classes are immutable.
What is Enum in Java?
Enum is used to provide user defined type.
Enum is used to provide user defined type.
What is Java Annotations?
Java Annotations provide metadata. Introduced in Java5, from Java8 we can have repeated annotations.
Java Annotations provide metadata. Introduced in Java5, from Java8 we can have repeated annotations.
What is Java Reflection API? Why it’s so important to have?
Reflection is the ability to inspect the code and dynamically modify or call it during runtime. It's very useful and used heavily in lots of framework like jUnit, spring, hibernate,etc.
Reflection is the ability to inspect the code and dynamically modify or call it during runtime. It's very useful and used heavily in lots of framework like jUnit, spring, hibernate,etc.
What is composition in java?
Composition refers to 'has a' behaviour. If a class contains instance of another class, it is called composition. Composition should always be preferred over inheritance.
What is the benefit of Composition over Inheritance?
Composition is more flexible than inheritance and leads to better maintainable code. In case of inheritance it's impossible to change code in the base class. So, it leads to rigid system. Whereas in composition I can easily change code.
Composition refers to 'has a' behaviour. If a class contains instance of another class, it is called composition. Composition should always be preferred over inheritance.
What is the benefit of Composition over Inheritance?
Composition is more flexible than inheritance and leads to better maintainable code. In case of inheritance it's impossible to change code in the base class. So, it leads to rigid system. Whereas in composition I can easily change code.
How to sort a collection of custom Objects in Java?
We can sort a collection of custom Objects by implementing Comparable or Comparator interface.
Comparable has one method, int compareTo(Object o) which has to be implemented to provide the sorting order. Comparable is used to provide natural ordering.
Comparator has also one method, int compare(Object o1,Object o2), we can define any number of comparators for an object.
After this we can use Arrays.sort() or Collections.sort() methods to sort arrays and collections by natural order or by using any comparator.
We can sort a collection of custom Objects by implementing Comparable or Comparator interface.
Comparable has one method, int compareTo(Object o) which has to be implemented to provide the sorting order. Comparable is used to provide natural ordering.
Comparator has also one method, int compare(Object o1,Object o2), we can define any number of comparators for an object.
After this we can use Arrays.sort() or Collections.sort() methods to sort arrays and collections by natural order or by using any comparator.
What is inner class in java? Why is it required? Give example.
In java, a class can contains other classes. These classes can be static or non-static. Static classes are called nested classes and others are called inner classes.
These are required for providing encapsulation.
For creating a LinkedList, we can define Node as inner class. HashMap uses static nested class Entry.
In java, a class can contains other classes. These classes can be static or non-static. Static classes are called nested classes and others are called inner classes.
These are required for providing encapsulation.
For creating a LinkedList, we can define Node as inner class. HashMap uses static nested class Entry.
- Inner classes in Java
Inner class example:
class Outer { private int outer_var=10; private static int outer_static_var=55; //Inner class class Inner{ int inner_var=20; void inner_method(){ System.out.println("outer private var from inner:"+outer_var); } } //Outer class void outer_method(){ // System.out.println("Can't access inner class variables"+ inner_var); //throws compiler error System.out.println("outer method"); } //Method Local Inner class void methodLocalInnerClass(){ final int method_var=11; //notice final keyword class MethodLocal{ int method_local_var=30; void methodLocalClassMethod(){ System.out.println("From method local inner class:"+ method_var); // can access only final variables } } //This class can be accessed only inside this method MethodLocal methodLocal = new MethodLocal(); methodLocal.methodLocalClassMethod(); } //Static nested class static class InnerStatic{ int static_class_var=10; void static_class_method(){ //can access only static variables of outer class System.out.println("Hello from method from static nested class:"+ outer_static_var ); System.out.println("Can contain non static varialbes also:"+static_class_var); } } } public class JavaInnerClasses{ public static void main(String[] args) { //Outer class Outer outer = new Outer(); outer.outer_method(); //Inner class Outer.Inner inner = outer.new Inner(); inner.inner_method(); //Method Local Inner class outer.methodLocalInnerClass(); //Anonymous inner class Thread t = new Thread(new Runnable() { @Override public void run() { System.out.println("Hello from method of anonymous inner class"); } }); t.start(); //Static nested class Outer.InnerStatic innerStatic = new Outer.InnerStatic(); innerStatic.static_class_method(); } }
What is anonymous inner class? Why they are used?
An inner class without a name is anonymous inner class. They make code concise and less number of classes are created thus reducing complexity.
An inner class without a name is anonymous inner class. They make code concise and less number of classes are created thus reducing complexity.
What is Classloader in Java?What are different types of classloaders?
Classloader loads classes in Java. There are three different type of classloaders and they are in parent child heirarchy. Each classloader can see only the classes loaded by it's children. If a class isn't loaded it delegates the task to it's parent.(?) The three types of classloaders are:
1) Bootstrap: It loads the classes at JVM startup. Loads rt.jar(?)
2) System: It loads the system classes. Loads lib/ext classes(?)
3) Extension: This can be extended by user to create custome classloader.
Classloader loads classes in Java. There are three different type of classloaders and they are in parent child heirarchy. Each classloader can see only the classes loaded by it's children. If a class isn't loaded it delegates the task to it's parent.(?) The three types of classloaders are:
1) Bootstrap: It loads the classes at JVM startup. Loads rt.jar(?)
2) System: It loads the system classes. Loads lib/ext classes(?)
3) Extension: This can be extended by user to create custome classloader.
What is ternary operator in java?
It's a shortcut to write if else condition. It's syntax is (condition)?(expression1):(expression2) , if condition is true , expression is evaluated else expression2 is evaluated.
What does super keyword do?
suepr keyword is used to refer to the superclass object.
What is break and continue statement?
break and conitue both are used inside loop , break terminates the loop and continue carries forward to the next iteration.
What is this keyword?
this refers to the currently executing object.
What is default constructor?
If user doesn't provide a constructor then JVM inserts a default no arg constructor in the class.
Can we have try without catch block?
Yes, we can have try with finally only.
What is Garbage Collection?
It's the process of automatic reclaiming memory from the objects which are no longer referenced.
What is Serialization and Deserialization?
Serialization is the process writing an object state in binary format and deserialization is the process of reading that binary format and converting it back to the original object.
The terms are also used for converting object to json/xml format and vice versa.
What is the use of System class?
System class provides many utility functions such as properties, copy ,etc. and reference to objects such as out, calendar, runtime, etc.
It's a shortcut to write if else condition. It's syntax is (condition)?(expression1):(expression2) , if condition is true , expression is evaluated else expression2 is evaluated.
What does super keyword do?
suepr keyword is used to refer to the superclass object.
What is break and continue statement?
break and conitue both are used inside loop , break terminates the loop and continue carries forward to the next iteration.
What is this keyword?
this refers to the currently executing object.
What is default constructor?
If user doesn't provide a constructor then JVM inserts a default no arg constructor in the class.
Can we have try without catch block?
Yes, we can have try with finally only.
What is Garbage Collection?
It's the process of automatic reclaiming memory from the objects which are no longer referenced.
What is Serialization and Deserialization?
Serialization is the process writing an object state in binary format and deserialization is the process of reading that binary format and converting it back to the original object.
The terms are also used for converting object to json/xml format and vice versa.
What is the use of System class?
System class provides many utility functions such as properties, copy ,etc. and reference to objects such as out, calendar, runtime, etc.
What is instanceof keyword?
instancof keyword is used to check the type of a reference.
instancof keyword is used to check the type of a reference.
Can we use String with switch case?
Yes , from Java7 onwards.
What is the difference in String implementation in various Java versions?
substring method implementation changed in 7, now big strings are not prevented from gc.
Java is Pass by Value or Pass by Reference?
Java is strictly Pass by Value, even the reference is passed by value.
Yes , from Java7 onwards.
What is the difference in String implementation in various Java versions?
substring method implementation changed in 7, now big strings are not prevented from gc.
Java is Pass by Value or Pass by Reference?
Java is strictly Pass by Value, even the reference is passed by value.
What is difference between Heap and Stack Memory?
In java, main memory has been divided into two main categories, heap and stack. All the memory except local variables is allocated on heap. Local variables and function calls goes to stack.
In java, main memory has been divided into two main categories, heap and stack. All the memory except local variables is allocated on heap. Local variables and function calls goes to stack.
Write thread safe singleton.
Enum is the best way to write singleton. It's threadsafe as well as provide protection from Reflection also. Earlier double checked singleton was used. Bill Pugh (????????)
Enum is the best way to write singleton. It's threadsafe as well as provide protection from Reflection also. Earlier double checked singleton was used. Bill Pugh (????????)
enum EnumSinleton { Instance; public void doSomething(){ System.out.println("Hello World!"); } } //Double checked Singleton class Singleton{ private static Singleton instance; public Singleton() {} public static Singleton getInstance(){ if(instance==null){ synchronized (Singleton.class) { if(instance==null){ instance=new Singleton(); } } } return instance; } } public class SingletonDemo { public static void main(String[] args) { EnumSinleton.Instance.doSomething(); } }Write code to solve producer consumer problem.
import java.util.LinkedList; import java.util.Queue; class Producer implements Runnable{ private Queue<Integer> queue; private int size; Producer(Queue<Integer> queue,int size){ this.queue=queue; this.size=size; } @Override public void run() { for(int i=1;i<=10;i++){ try { System.out.println("Produced:"+produce(i)); Thread.sleep(400); } catch (InterruptedException e) { e.printStackTrace(); } } } private int produce(int i) throws InterruptedException{ synchronized (queue) { while(queue.size()==size){ queue.wait(); } queue.add(i); queue.notify(); return i; } } } class Consumer implements Runnable{ private Queue<Integer> queue; private int size; Consumer(Queue<Integer> queue,int size){ this.queue=queue; this.size=size; } @Override public void run() { while(true){ try { System.out.println("Consumed:"+consume()); Thread.sleep(800); } catch (InterruptedException e) { e.printStackTrace(); } } } private int consume() throws InterruptedException{ synchronized (queue) { while(queue.isEmpty()){ queue.wait(); } queue.notify(); return queue.remove(); } } } public class ProducerConsumerDemo { public static void main(String[] args) { Queue<Integer> queue = new LinkedList<>(); int size = 3; Thread producer = new Thread(new Producer(queue, size)); Thread consumer = new Thread(new Consumer(queue,size)); producer.start(); consumer.start(); } }
How to create a thread? Which way do you prefer? What's the difference between runnable and callable?
Thread can be created in three ways.a) By extending Threadb) By implementing Runnable interface and passing it's object to Thread constructor.c) By implementing Callable interface and passing it's object to Executor.submit() method.
import java.util.concurrent.Callable; import java.util.concurrent.ExecutionException; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.concurrent.Future; import java.util.concurrent.TimeUnit; class Thread1 extends Thread{ @Override public void run(){ System.out.println("Thread created by extending Thread class"); } } class Thread2 implements Runnable{ @Override public void run() { System.out.println("Thread created by implementing runnable"); } } class Thread3 implements Callable<Integer>{ @Override public Integer call() throws Exception { System.out.println("Thread created by implementing callable"); return 1; } } public class ThreadDemo { public static void main(String[] args) { Thread t1 = new Thread1(); Thread t2 = new Thread(new Thread2()); t1.start(); t2.start(); ExecutorService executorService = Executors.newFixedThreadPool(1); Future<Integer> future= executorService.submit(new Thread3()); try { System.out.println("Result of callable:"+future.get()); } catch (InterruptedException | ExecutionException e) { e.printStackTrace(); } executorService.shutdown(); while(executorService.isTerminated()){ try { executorService.awaitTermination(100,TimeUnit.MILLISECONDS); } catch (InterruptedException e) { e.printStackTrace(); } } } }
What is volatile keyword?
Volatile keyword can be used with a variable. It provides happens-before guarantee. In a multi-threaded application, all the threads will always read the value of volatile variable from main memory. Thus, a subsequent read of a volatile variable after a write is guaranteed to show the latest updated value. In fact, all the variables which were visible to the thread at the writing time will be visible to the thread reading the volatile variable.
String created as new creates two objects, one on heap and one in string pool whereas string created as literal creates only object in string pool.
What does intern method of String does?
It moves the string to string pool(if required) and returns a reference of it else returns the reference of a string which is already in string pool.
Why wait, notify, notifyAll are declared in Object class? Why wait and notify should be called from synchronized block? notify vs notifyAll?
Wait, notify and notifyAll are called on objects. Hence, they have to be defined in Object class.
Wait and notify should be called from synchronized block to ensure correct communication. Let's say a thread check a condition before calling wait and found the condition true, however condition might change by the time the thread calls wait. Similar, logic for notify. Wait should be called inside while loop to prevent spurious wake up calls.Nofiy notifies a single randomly chosen thread. NofiyAll notifies all the waiting threads. After that JVM choses a single thread based on it's scheduler.
What will be the output of following code?
package general; public class WaitNotifyOnString { public static void main(String[] args) throws Exception { String str="str"; synchronized (str) { System.out.println("1"); str+="1"; //Line 1 str.wait(); //Line 2 System.out.println("2"); } } }*
IlegalMonitorStateException because the object on which lock is acquired has been changed. If we comment line 1, it will print 1 and wait indefinitely.
What is the difference between collection, Collection and Collections?
collection refers to a group of objects.
Collection is the base interface of Collection framework.
Collections is a utility class for common operation in Collection framework.
collection refers to a group of objects.
Collection is the base interface of Collection framework.
Collections is a utility class for common operation in Collection framework.
What are the importatnt interfaces of collections framework? Draw the diagram for collections framework heirarchy.
Important interfaces of collections framework are List,Set,Map,Queue,SortedSet,SortedMap,NavigableList,NavigableMap,etc.
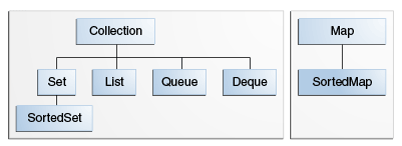
Here is brief heirarchy:
Here is the complete heirarchy:
Important interfaces of collections framework are List,Set,Map,Queue,SortedSet,SortedMap,NavigableList,NavigableMap,etc.
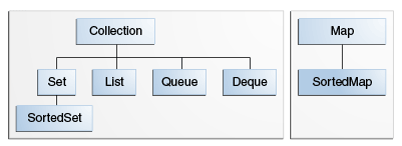
Here is brief heirarchy:
Here is the complete heirarchy:
How hashmap, hashset and treemap works? What is the difference between HashMap, Hashtable, concurrenthashmap?
Hashmap contains a static nested class called Entry which contains key and value object. HashMap maintains an array of linkedlist of Entry object. When a new key-value pair is added to HashMap, it calculates it uses it's hashcode to determine the index of the array. After that it iterates through the linkedlist and uses the equals method to check whether the key already exists, if it finds the key it updates the value with the new value else it appends the entry object to the linkedlist.
HashSet internally uses hashmap. Here, each key is mapped to a object. If the hashmap contains the key set doesn't add it.
TreeMap uses Red-Black tree which is a balanced binary tree and provides O(log n) search time. Regarding treemap it's important to note that spaces sort before characters and capital sort before small letters.
Hashtable is exactly similar to hashmap except that it's a synchronized class i.e, all the methods of Hashtable are synchronized. Also, hashtable doesn't allow null key and values while hashmap allows one null key and multiple null values. Earlier Hashtable used to implement Dictionary interface, later it was retrofitted by implementing Map interface.
ConcurrentHashMap is also synchronized but it's more efficient thatn Hashtable because it doesn't lock the entire Map and locks only portions of it, thus increasing the performance.
Hashmap contains a static nested class called Entry which contains key and value object. HashMap maintains an array of linkedlist of Entry object. When a new key-value pair is added to HashMap, it calculates it uses it's hashcode to determine the index of the array. After that it iterates through the linkedlist and uses the equals method to check whether the key already exists, if it finds the key it updates the value with the new value else it appends the entry object to the linkedlist.
HashSet internally uses hashmap. Here, each key is mapped to a object. If the hashmap contains the key set doesn't add it.
TreeMap uses Red-Black tree which is a balanced binary tree and provides O(log n) search time. Regarding treemap it's important to note that spaces sort before characters and capital sort before small letters.
Hashtable is exactly similar to hashmap except that it's a synchronized class i.e, all the methods of Hashtable are synchronized. Also, hashtable doesn't allow null key and values while hashmap allows one null key and multiple null values. Earlier Hashtable used to implement Dictionary interface, later it was retrofitted by implementing Map interface.
ConcurrentHashMap is also synchronized but it's more efficient thatn Hashtable because it doesn't lock the entire Map and locks only portions of it, thus increasing the performance.
Which two interfaces are used for providing sorting order?
Comparable and Comparator interfaces are used to provide sorting order.
Comparable provides natural ordering. It has a single method compareTo which accepts an object as parameter, based on comparison it returns an integer which can be -ve,+ve or zero.
Comparator also provides a single method compare which accepts two objects as parameters to compare and based on that returns either a +ve,-ve or zero value. Thus, a number of comparator objects can be used for providing different types of sorting orders.
Example Code:
import java.util.Arrays; import java.util.Collections; import java.util.Comparator; import java.util.List; class Employee implements Comparable<Employee>{ private String name; private int age; private int salary; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public int getSalary() { return salary; } public void setSalary(int salary) { this.salary = salary; } Employee(String name,int age,int salary){ this.name=name; this.age=age; this.salary=salary; } @Override public int compareTo(Employee o) { return this.name.compareTo(o.getName()); } @Override public String toString(){ return name; } } class AgeComparator implements Comparator<Employee>{ @Override public int compare(Employee o1, Employee o2) { if(o1.getAge()<o2.getAge()) return -1; else if(o1.getAge()>o2.getAge()) return 1; else return 0; } } class SalaryComparator implements Comparator<Employee>{ @Override public int compare(Employee o1, Employee o2) { return (Integer.valueOf(o1.getSalary()).compareTo(Integer.valueOf(o2.getSalary()))); } } public class SortingDemo { public static void main(String[] args) { Employee praveen= new Employee("Praveen",27, 2000); Employee manjesh = new Employee("Manjesh", 30, 5000); Employee rakesh = new Employee("Rakesh", 33, 10000); List<Employee> employees = Arrays.asList(praveen,manjesh,rakesh); //Sorting on natural ordery by Comparable interface Collections.sort(employees);//will accept only those who extend Comparable System.out.println("Based on natural ordering(names):"+ employees); Collections.sort(employees,new AgeComparator()); System.out.println("Based on age comparator :"+ employees); Collections.sort(employees,new SalaryComparator()); System.out.println("Based on salary comparator :"+ employees); } }
What are multithreading best practices?
1) Try to use as much as possible immutable classes.
2) Never rely on order of threads.
3) Don't use synchronized keyword on methods. Use them in critical block.
4) Always call wait method in while loop.
5) Use thread pool. Use third party library instead of reinventing the wheel.
6) Prefer callable over runnable.
7) Prefer concurrent classes over synchronized classes.
8)Prefer lock over synchronized keyword.
9)Use local variables.
10) Avoid static variables.
How threads are scheduled in java?
Threads are scheduled in java by JVM. Thus, different JVM can produce different order of JVM, JVM may schedule the threads by itself or it can map them to the underlying OS. Usually, they are implemented as native thread and mapped to the underlying OS. In case that's not supported there is fallback mechanisim of green threads too.
What are the various map implementations?
Java has following map implementations:
HashMap, LinkedHashMap, Hashtable, ConcurrentHashMap, EnumMap, WeakHashMap,
IdentityHashMap
Abstraction vs Encapsulation?
Abstraction and Encapsulation are quite similar as they both hide complexity of a system.
Abstraction and Encapsulation are quite similar as they both hide complexity of a system.
However, abstraction is at a higher level than encapsulation. Abstraction leaves out all the details and focus on the high level picture. Encapsulation is implementation of abstraction(??). It hides the implementation details. It also binds together the data and code.
In java, abstraction is achieved by using interfaces and abstract classes and encapsulation is achieved by mandatory use of class and access specifiers public,private and protected.
Countdownlatch vs Cyclicbarrier?
Both are used for synchronization among threads, however Cyclicbarrier can be reused but countdownlatch can't be reused.
Both are used for synchronization among threads, however Cyclicbarrier can be reused but countdownlatch can't be reused.
Can a class be abstract and final?
No, a class marked as abstract is useful only when it's extended and abstract functinons are given implementatins whereas final means a class can't be exntended. Thus, there is contradiction, hence a class can't be marked as abstract and final both.
No, a class marked as abstract is useful only when it's extended and abstract functinons are given implementatins whereas final means a class can't be exntended. Thus, there is contradiction, hence a class can't be marked as abstract and final both.
Can hashmap leads to race condition and inifinte loop in get method?
Yes, if multiple threads are using same hashmap, it can lead to race condition while map resizing and get function may enter infinite loop.(explain in detail..)
How to stop a thread?
Best way to stop a thread is to use some boolean as condition and check it inside the thread, when the work is finished, set the boolean to false.
Earlier there were stop method but now it has been deprecated because it may cause deadlock and it's not safe to use.
What is a collection? What is collections framework ? What are the benefits ?
A collection is a group of objects.
Java provides a lot of classes and utility functions to work on various collections. It's so vast and useful that it's called a framework.
Benefits:
1) Reduces development time.
2) Improves performance
3) Improves code quality
4) Uses generics which provide compile time safety and saves us from casting objects.
5) Collections are dynamic.
What is the difference between ordered and sorted?
Order is based on order we have put an element into a collection. It doesn't depend on the values of elements.
Sorted collection is sorted based on some values of the elements.
What is static binding and dynamic binding? Difference?
OR
What is compile time binding and runtime binding?
Static binding is the binding of methods to the references at compile time whereas dynamic binding is when the methods are resolved at runtime based on the object type. Dynamic binding happens in case of polymorphism/overriding while static binding happens in rest of the cases including overloading.
Give real life examples of abstraction,encapsulation,inheritance.
A car is an abstraction. It's an entity which provide us an interface to transport ourselves. There is handle, gear, key, and a few other functions. However, we never buy a car. We buy a specific model of it, car is an abstract idea here. Encapsulation is what hides the inner workings of car like how the engine works, etc.
Polymorphism can also be seen in car, depending on which gear the car is, the car runs on that gear.
Take example of Phone/Mobile and explain in detail.......
How to create a jar file?How to run a JAR file through command prompt?
To create jar of HelloWorld.java , first compile it using javac HelloWorld.java, then execute
jar cfe myJar.jar HelloWorld HelloWorld.class
here e is for entry point which is HelloWorld
To execute : java -jar myJar.jar
if it is not able to find main class , put current directory in classpath by executing:
java -cp . -jar myJar.jar
What is the use of System class?
System class provides many utility functions e.g to print to console, copy arrays, garbage collection, get system properties, etc.
What is instanceof keyword?
instanceof keyword is used to check whether a ref is of some type.
Can we use String with switch case?
From Java7 onwards, yes.
Java is Pass by Value or Pass by Reference?
Java is strictly pass by reference. Even the reference is passed by value.
What is difference between Heap and Stack Memory?
Stack memory contains only local variables and method calls, rest goes to heap such as instance variables, objects.
Class vs Object?
Class is the blueprint to create objects while Object is an instance of that blueprint.
What are the rules of overriding?
1. The overriding method can't reduce the visibility of the super class method.
2. The overriding method can't throw broader exceptions.
Is java platform independent? How?
Yes, java compiler compiles code to an intermediate representation which is then executed by JVM which is platform specific. Thus, java code can run on any platform.
What is volatile keyword?
Volatile keyword can be used only with variables, it guarantees a happens before relationship. A write on volatile by a thread will be visible to all the subsequent reads by any thread. In fact, all the variables values seen by the writing thread will be seen to other threads. Thus, volatile acts like a synchronized block.
Volatile keyword can be used only with variables, it guarantees a happens before relationship. A write on volatile by a thread will be visible to all the subsequent reads by any thread. In fact, all the variables values seen by the writing thread will be seen to other threads. Thus, volatile acts like a synchronized block.
Why wait, notify, notifyAll are declared in Object class? Why wait and notify should be called from synchronized block? notify vs notifyAll?
A thread wait, notify or notifyAll on an object. Thus, they have to be defined in Object class. Wait and notify should be called from synchronized block otherwise there will be IllegalMonitorStateException, it is made so that wait and notify happens correctly, because a thread wait or notify after checking some condition, thus this code should go into synchronized block otherwise wrong notify or wait could happen. Notify notifies a random thread waiting on the object and notifyAll notifies all the waiting threads, after which JVM choses a thread to run.
Thread lifecycle
Thread has five states: New, Runnable, Running, Waiting/Blocked, Dead.
A thread just created and before calling start() is in New state. After calling start(), it enters runnable state and is considere alive. A thread can't be started twice, it will cause IllegalMonitorStateException to be thrown(?). A thread might go into waiting/blocked by calling sleep() or wait(). After finishing it's task a thread is dead. A dead thread can't be started again.
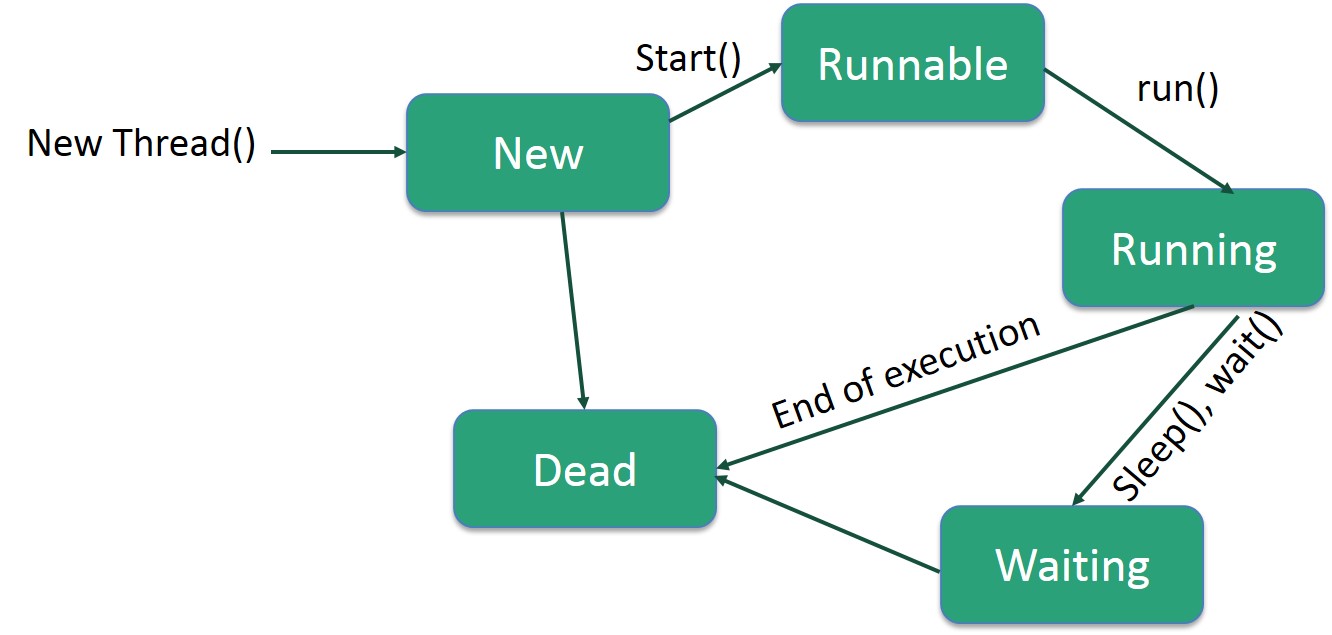
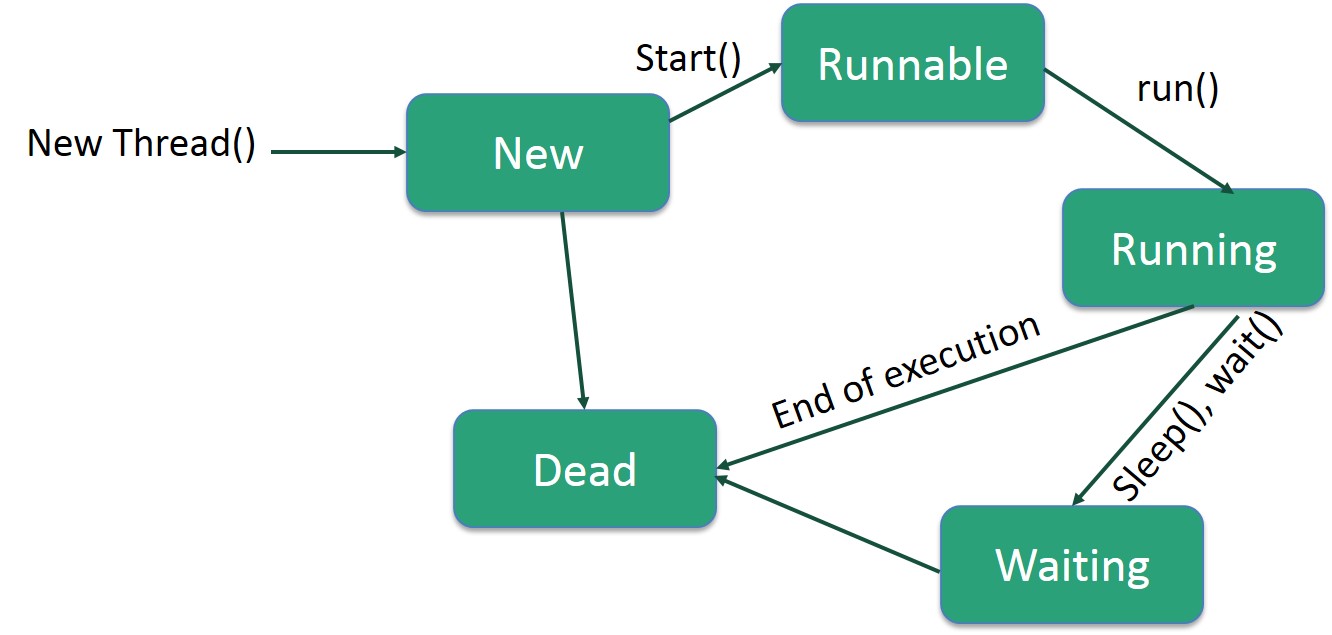
throw vs throws?
throw keyword is used to throw some exception or error. If it's a checked exception it must be handled by the method itself.
throws keyword is used with method to indicate that this method throws exception. If it's a checked exception(which most of the time is) it must be handled by the calling method.
Error vs exception?
Error is a fatal situation from which a program can't recover and it should terminate. e.g StackOverflowError, JVMOutofMemoryError, etc.
Exception is an undesired situation in a program flow from which program may recover. e.g FileNotFoundException, IOException, etc.
What is composition, aggregation and association?
Association: Both objects exist independently. No ownership. 'Has a' relationship is not there.
Aggregation: Both objects exist independently. Ownership is there. Child class can't belong to any other parent object(??) 'Has a' relationship.
Composition: Strong aggregation. Child object can't exist without parent. Ownership is there obviously.
ReadWriteLock
Only two methods, readLock , writeLock ,
readLock can be held simultaneously by mulitple threads
while writeLock is mutually exclusive.
Reentrant vs synchronized
Both are same, but rentrant provides lot more functionalites such as on conditions, get count of threads waiting, try lock, etc.
Lock heirarchy

Why map is not part of collections framework?
Map is not a collection, it's a key value pair. Also, collections common operations like add, remove doesn't apply to map.
Only two methods, readLock , writeLock ,
readLock can be held simultaneously by mulitple threads
while writeLock is mutually exclusive.
Reentrant vs synchronized
Both are same, but rentrant provides lot more functionalites such as on conditions, get count of threads waiting, try lock, etc.
Lock heirarchy
Why map is not part of collections framework?
Map is not a collection, it's a key value pair. Also, collections common operations like add, remove doesn't apply to map.
What Collection doesn't implement Serializable and Cloneable interface?
It's the responsibility of the implementing class.
It's the responsibility of the implementing class.
What is the difference between fail fast and fail safe iterator?
Fail fast iterator like iterator of ArrayList throws exception(ConcurrentModificationException) when the underlying collection changes while fail safe doesn't e.g, iterator of CopyOnWriteArrayList
Fail fast iterator like iterator of ArrayList throws exception(ConcurrentModificationException) when the underlying collection changes while fail safe doesn't e.g, iterator of CopyOnWriteArrayList
What is copyonwritearraylist and why it is used?
It is in util.concurrency package, it maintains a copy of arraylist and returns it, it provides fail safe iterator.
It is in util.concurrency package, it maintains a copy of arraylist and returns it, it provides fail safe iterator.
What is thread pool? How to use, why to use?
What is Reentrant lock?
What is fork join framework?
How to ensure sequence in multiple threads
start vs run
What is Java memory model ?
What is thread group? Should we use it ?
What is green thread?
The threads that are scheduled by a runtime library or virtual machine (VM) instead of natively by the underlying operating system. Green threads emulate multithreaded environments without relying on any native OS capabilities, and they are managed in user space instead of kernel space, enabling them to work in environments that do not have native thread support.
Whole method synchronized vs critical section synchronized ?
Synchronized block because synchronized has considerable overhead and having synchronized where it's not required will slow down the application.
IF a method throws FileNotFoundException in super , can the same method in subclass throws IOException?
No, can't throw broader exceptions for checked exceptions.
If a method throws NullPointerException in super, can the same method in subclass throws ArrayStoreException
Yes, for unchecked exception no issues
Synchronized block because synchronized has considerable overhead and having synchronized where it's not required will slow down the application.
IF a method throws FileNotFoundException in super , can the same method in subclass throws IOException?
No, can't throw broader exceptions for checked exceptions.
If a method throws NullPointerException in super, can the same method in subclass throws ArrayStoreException
Yes, for unchecked exception no issues
Can you access non static variable in static context?Why or why not ?
No, because static is related to class and non static is related to instance, which instance value will be used!
No, because static is related to class and non static is related to instance, which instance value will be used!
What is the base class of all exceptions?
Throwable
Throwable
Is empty java source file valid?
Yes
Can I print something from a program not having main method?
Prior to Java7 print from static block. Java7 onwards you can't execute a java program not having main method.
Why main method is static?
So that JVM doesn't has to create object of class, it it has to there will be confusion which object to create(?) why not create and discard(??)
I have two methods of same name, one accepts string, other object, I pass argument as null, which one will be called?
String one, the most specific is always called.
I have two methods of same name, one accepts string, other stringbuilder, I pass argument as null, which one will be called?
Yes
Can I print something from a program not having main method?
Prior to Java7 print from static block. Java7 onwards you can't execute a java program not having main method.
Why main method is static?
So that JVM doesn't has to create object of class, it it has to there will be confusion which object to create(?) why not create and discard(??)
I have two methods of same name, one accepts string, other object, I pass argument as null, which one will be called?
String one, the most specific is always called.
I have two methods of same name, one accepts string, other stringbuilder, I pass argument as null, which one will be called?
Compiler error, ambiguous situation here.
What is bytecode?
Intermediate representation of java source code.
Intermediate representation of java source code.
What are the features of Java language?
Platform independent
Architecture Neutral
Interpreted and compiled
Strongly typed
Simple
Robust
Automatic garbage collection
Constructor vs method?
Is constructor inherited?
No
Can constructor be overloaded?
Yes
can constructor be overridden?
No, because they aren't inherited.
does java provides copy constructor?
what is the first statement of any constructor?
this or super.
Explain how memory is allocated by JVM?
How is java WORA?
Java is Write Once, Run Anywhere by use of bytecode. Java compiler compiles java source code to bytecode which is then executed by JVM which is specific to each OS.
Can an exception be rethrown?
Yes
Platform independent
Architecture Neutral
Interpreted and compiled
Strongly typed
Simple
Robust
Automatic garbage collection
Constructor vs method?
Constructor | Method |
---|---|
Name same as class | Name can be anything. |
Can't return anything | Can return anything including void |
Can't call directly, called only during object creation |
Can be called any number of times |
Is constructor inherited?
No
Can constructor be overloaded?
Yes
can constructor be overridden?
No, because they aren't inherited.
does java provides copy constructor?
what is the first statement of any constructor?
this or super.
Explain how memory is allocated by JVM?
How is java WORA?
Java is Write Once, Run Anywhere by use of bytecode. Java compiler compiles java source code to bytecode which is then executed by JVM which is specific to each OS.
Can an exception be rethrown?
Yes
What is a thread ? What is a process ? What's the difference ?
A thread is a unit of process(?). A process is a program in execution.
Differences:
All threads same the heap of parent process and has it's own stack while each process has it's own heap and stack.
Threads are lightweight , so take up less resources and the overhead of context switch is much lesser compared to process.
Inter thread communication can be done easily as they share same heap while inter thread process communication has to be done via external mechanism.
A developer has more control over thread scheduling compared to process scheduling.(?)
How to create a thread ? Which way do you prefer ? What's the difference between runnable and callable ?
There are mainly two ways of creating threads :
a) By extending Thread class :
b) By implementing Runnable or Callable interface:
I prefer implementing Runnable interface because it's leave my class free to extend any other class. Also , there is no point of extending Thread class as we are not specializing it's behaviour.
A thread is a unit of process(?). A process is a program in execution.
Differences:
All threads same the heap of parent process and has it's own stack while each process has it's own heap and stack.
Threads are lightweight , so take up less resources and the overhead of context switch is much lesser compared to process.
Inter thread communication can be done easily as they share same heap while inter thread process communication has to be done via external mechanism.
A developer has more control over thread scheduling compared to process scheduling.(?)
How to create a thread ? Which way do you prefer ? What's the difference between runnable and callable ?
There are mainly two ways of creating threads :
a) By extending Thread class :
b) By implementing Runnable or Callable interface:
I prefer implementing Runnable interface because it's leave my class free to extend any other class. Also , there is no point of extending Thread class as we are not specializing it's behaviour.
What are multi-threading best practices ?
a) Don't synchronize entire methods , just synchronize the critical blocks.
b) Don't rely on the order of execution of statements. There is no guarantee.
c) Don't rely on using synchronized classes such as Hashtable and Vector , instead use non sychronized classes and synchronize their methods yourselves.
d) Use immutable classes as much as possible.
How to use Stack in Java?
Use Dequeue interface and LinkedList implementation. Dequeue has push,pop and peek methods.
Don't use Stack as it extends Vector class and it's methods are synchronized.
What is volatile keyword ?
Volatile keyword provides happens before guarantee. It can be used only with instance variables. A volatile variable value is always read from the main memory by all the threads and isn't cached.
What is thread safety ? What is race condition? What is deadlock ? What are the conditions for deadlock ? Give an example of deadlock. How to prevent deadlock ? What is livelock ?
Thread safety means program will work as expected. Race condition occurs when multiple threads compete to modify same resources. Deadlock is a situation when multiple threads are waiting for resources held by each other resulting in an infinite waiting. Four conditions for deadlock to happen :
a) Mutual exclusion:
b) Non preemptive behaviour:
c) Cyclic dependency
d) Hold and wait (?)
Semaphore vs Binary Semaphore vs mutex vs lock vs synchronized
Semaphore is used for counting threads which have reached a certain breakpoint before taking any action.
Binary semaphore is same as mutex.
Mutex is a mechanism to provide synchronization.
Difference between mutex and semaphore is of ownership , mutex owns the resource whereas semaphore doesn't.
Lock is used to obtain lock over a resource , lock is same as mutex except it provides more flexibility such as read write locks.
Synchronized keyword is same as obtaining a lock and synchronizing the code.
Why wait , notify , notifyAll are declared in Object class ? Why wait and notify should be called from synchronized block and why in an infinite loop? notify vs notifyAll ?
Wait , notify and notifyAll are declared in Object class because they can be called only by the thread holding a lock on an object.
Wait and notify should be called from synchronized block because they can be called only after achieving lock on an object. It's a good practice to call them inside infinite loop because sometimes there are spurious wake-up calls.
Notify will notify a randomly selected waiting thread while notify all will notify all the waiting threads , after which JVM will select a thread to execute.
How to create immutable objects in java ?
To create immutable objects :
a) Mark the class as final.
b) Make all instance variables final and private , don't provide any setters.
c) If there is any mutable object, make that class immutable.
d)If it is exposing any list return Collections.unmodifiableList() and similarly other collections.
a) Don't synchronize entire methods , just synchronize the critical blocks.
b) Don't rely on the order of execution of statements. There is no guarantee.
c) Don't rely on using synchronized classes such as Hashtable and Vector , instead use non sychronized classes and synchronize their methods yourselves.
d) Use immutable classes as much as possible.
How to use Stack in Java?
Use Dequeue interface and LinkedList implementation. Dequeue has push,pop and peek methods.
Don't use Stack as it extends Vector class and it's methods are synchronized.
What is volatile keyword ?
Volatile keyword provides happens before guarantee. It can be used only with instance variables. A volatile variable value is always read from the main memory by all the threads and isn't cached.
What is thread safety ? What is race condition? What is deadlock ? What are the conditions for deadlock ? Give an example of deadlock. How to prevent deadlock ? What is livelock ?
Thread safety means program will work as expected. Race condition occurs when multiple threads compete to modify same resources. Deadlock is a situation when multiple threads are waiting for resources held by each other resulting in an infinite waiting. Four conditions for deadlock to happen :
a) Mutual exclusion:
b) Non preemptive behaviour:
c) Cyclic dependency
d) Hold and wait (?)
Semaphore vs Binary Semaphore vs mutex vs lock vs synchronized
Semaphore is used for counting threads which have reached a certain breakpoint before taking any action.
Binary semaphore is same as mutex.
Mutex is a mechanism to provide synchronization.
Difference between mutex and semaphore is of ownership , mutex owns the resource whereas semaphore doesn't.
Lock is used to obtain lock over a resource , lock is same as mutex except it provides more flexibility such as read write locks.
Synchronized keyword is same as obtaining a lock and synchronizing the code.
Why wait , notify , notifyAll are declared in Object class ? Why wait and notify should be called from synchronized block and why in an infinite loop? notify vs notifyAll ?
Wait , notify and notifyAll are declared in Object class because they can be called only by the thread holding a lock on an object.
Wait and notify should be called from synchronized block because they can be called only after achieving lock on an object. It's a good practice to call them inside infinite loop because sometimes there are spurious wake-up calls.
Notify will notify a randomly selected waiting thread while notify all will notify all the waiting threads , after which JVM will select a thread to execute.
How to create immutable objects in java ?
To create immutable objects :
a) Mark the class as final.
b) Make all instance variables final and private , don't provide any setters.
c) If there is any mutable object, make that class immutable.
d)If it is exposing any list return Collections.unmodifiableList() and similarly other collections.
Sleep vs wait vs yeild
Sleep doesn't release the lock.
Wait releases the lock.
Yeild might release the lock
Sleep doesn't release the lock.
Wait releases the lock.
Yeild might release the lock
How threads are scheduled in java?
Threads are scheduled by JVM scheduler. Since JVM depends on underlying OS , so different JVM may have different scheduling algorithms. Sometimes , JVM may also map to the native OS.
What is Countdownlatch?
It is used to achieve synchronization among multiple threads e.g, in a multiple player game waiting for all the players to join the game.
Threads are scheduled by JVM scheduler. Since JVM depends on underlying OS , so different JVM may have different scheduling algorithms. Sometimes , JVM may also map to the native OS.
What is Countdownlatch?
It is used to achieve synchronization among multiple threads e.g, in a multiple player game waiting for all the players to join the game.
Countdownlatch vs Cyclicbarrier
Cyclicbarrier can be reused whereas Coundownlatch can't be reused.
How to stop a thread?(repeated?)
A thread should be stopped by using a boolean variable which should be changed to false on termination condition.
What is thread pool? How to use, why to use? Write code.
Thread objects consume a large amount of memory. So , if an application uses a lot of threads , there will be significant overhead of allocating and deallocating large amount of memory. So, a pool of threads can be used from where new threads can be picked up and upon completion returned back to the pool.
Another benefit of using thread pool is that each application has a threshold level , say a web server can server at most n requests , in case there are more than n request , the web server will stop responding. But if it has implemented fixed thread pool , it will continue serving requests , though slowly.
In Java, Executors framework provide thread pool which provides different types of implementation such as fixed thread pool , dynamic thread pool , single thread pool ,etc.
Cyclicbarrier can be reused whereas Coundownlatch can't be reused.
How to stop a thread?(repeated?)
A thread should be stopped by using a boolean variable which should be changed to false on termination condition.
What is thread pool? How to use, why to use? Write code.
Thread objects consume a large amount of memory. So , if an application uses a lot of threads , there will be significant overhead of allocating and deallocating large amount of memory. So, a pool of threads can be used from where new threads can be picked up and upon completion returned back to the pool.
Another benefit of using thread pool is that each application has a threshold level , say a web server can server at most n requests , in case there are more than n request , the web server will stop responding. But if it has implemented fixed thread pool , it will continue serving requests , though slowly.
In Java, Executors framework provide thread pool which provides different types of implementation such as fixed thread pool , dynamic thread pool , single thread pool ,etc.
Threadlocal ? Pros and cons ?
When threadlocal keyword is used with a variable, each thread keeps a copy of that value with itself. Threadlocal can be useful in certain scenarios where each thread has some local state. However , it's use is discouraged because it can cause sublte bugs (? How ?)
When threadlocal keyword is used with a variable, each thread keeps a copy of that value with itself. Threadlocal can be useful in certain scenarios where each thread has some local state. However , it's use is discouraged because it can cause sublte bugs (? How ?)
Submit vs execute in thread pool
Submit accept both runnable and callable while execute accepts only runnable.
Submit can throw exceptions and return result.
What is readwritelock?
ReadWriteLock provides better performance when there are multiple threads which are reading. Since reading needn't be exclusive , there can be multiple reader threads simultaneously.
What is reentrant lock?
A reentrant lock means if a thread has acquired lock on one object , it can enter other methods also which are also synchronized on the same lock.
Fork join framework
?
How to ensure sequence in multiple threads
Using join() method.
start vs run
start() method creates a new thread , if we use run() , no new thread will be created , it will be just like calling another method.
What is Java memory model ?
Java memory model describes the rules of how Java synchronization works. All the happens first rules and all.
What is thread group ? Pros and cons?
In java, certain threads can be grouped. Now, certain operations can be run on those threadgroups. It can be useful in debugging also a lot.
What is break and continue statement?
Break is used to end the loop.
Continue is used to jump to the next iteration.
Submit accept both runnable and callable while execute accepts only runnable.
Submit can throw exceptions and return result.
What is readwritelock?
ReadWriteLock provides better performance when there are multiple threads which are reading. Since reading needn't be exclusive , there can be multiple reader threads simultaneously.
What is reentrant lock?
A reentrant lock means if a thread has acquired lock on one object , it can enter other methods also which are also synchronized on the same lock.
Fork join framework
?
How to ensure sequence in multiple threads
Using join() method.
start vs run
start() method creates a new thread , if we use run() , no new thread will be created , it will be just like calling another method.
What is Java memory model ?
Java memory model describes the rules of how Java synchronization works. All the happens first rules and all.
What is thread group ? Pros and cons?
In java, certain threads can be grouped. Now, certain operations can be run on those threadgroups. It can be useful in debugging also a lot.
What is break and continue statement?
Break is used to end the loop.
Continue is used to jump to the next iteration.
this refers to the current executing object or instance.
What is default constructor?
When we don't create a constructor of a class, JVM implicitly creates a default constructor having no parameters. It's function is to initialize the object.
Can we have try without catch block?
Yes, we can have try block with finally.
What is Garbage Collection?(Repeated?)
What is Serialization and Deserialization?
To serialize an object means to convert its state to a byte stream so that the byte stream can be reverted back into a copy of the object. A Java object is serializable if its class or any of its superclasses implements either the java.io.Serializable interface or its subinterface, java.io.Externalizable. Deserialization is the process of converting the serialized form of an object back into a copy of the object.
What is the use of System class?
The System class contains several useful class fields and methods. It cannot be instantiated.
Among the facilities provided by the System class are standard input, standard output, and error output streams; access to externally defined properties and environment variables; a means of loading files and libraries; and a utility method for quickly copying a portion of an array.
What is instanceof keyword?
The java instanceof operator is used to test whether the object is an instance of the specified type (class or subclass or interface).
Stackoverflow
Can we use String with switch case?(Repeated?)
Java is Pass by Value or Pass by Reference?
Java is strictly pass by reference, even the reference are copied and then passed as parameter.
What is difference between Heap and Stack Memory?
Only local primitive variables and local references are stored on stack. Rest of the things are stored on heap. Each thread has it's own stack whereas heap is shared by all the threads.
Class vs Object?
A class is a blueprint which you use to create objects. An object is an instance of a class - it's a concrete 'thing' that you made using a specific class. So, 'object' and 'instance' are the same thing, but the word 'instance' indicates the relationship of an object to its class.
This is easy to understand if you look at an example. For example, suppose you have a class House. Your own house is an object and is an instance of class House. Your sister's house is another object (another instance of class House).
No comments:
Post a Comment